A generalization/specialization (gen/spec) diagram may be considered to be an enhanced class diagram. Sometimes it is necessary to separate out the generalizations from the specific instances. As we mentioned at the beginning of this chapter, a koala bear is part of a class of marsupials, which is part of a class of animals. Sometimes we need to distinguish whether a koala bear is an animal or a koala bear is a type of animal. Furthermore, a koala bear can be a stuffed toy animal. So we often need to clarify these subtleties.
Generalization
A generalization describes a relationship between a general kind of thing and a more specific kind of thing. This type of relationship is often described as an “is a” relationship. For example, a car is a vehicle and a truck is a vehicle. In this case, vehicle is the general thing, whereas car and truck are the more specific things. Generalization relationships are used for modeling class inheritance and specialization. A general class is sometimes called a superclass, base class, or parent class; a specialized class is called a subclass, derived class, or child class.
Inheritance
Several classes may have the same attributes and/or methods. When this occurs, a general class is created containing the common attributes and methods. The specialized class inherits or receives the attributes and methods of the general class. In addition, the specialized class has attributes and methods that are unique and only defined in the specialized class. Creating generalized classes and allowing the specialized class to inherit the attributes and methods helps to foster reuse, because the code is used many times. It also helps to maintain existing program code. This allows the analyst to define attributes and methods once but use them many times, in each inherited class.
One of the special features of the object-oriented approach is the creation and maintenance of large class libraries that are available in multiple languages. So, for instance, a programmer using Java, .NET, or C# will have access to a huge number of classes that have already been developed.
Polymorphism
Polymorphism (meaning many forms), or method overriding (not the same as method overloading), is the capability of an object-oriented program to have several versions of the same method with the same name within a superclass/subclass relationship. The subclass inherits a parent method but may add to it or modify it. The subclass may change the type of data, or change how the method works. For example, there might be a customer who receives an additional volume discount, and the method for calculating an order total is modified. The subclass method is said to override the superclass method.
When attributes or methods are defined more than once, the most specific one (the lowest in the class hierarchy) is used. The compiled program walks up the chain of classes, looking for methods.
Abstract Classes
Abstract classes are general classes and are used when gen/spec is included in the design. The general class becomes the abstract class. The abstract class has no direct objects or class instances, and is only used in conjunction with specialized classes. Abstract classes usually have attributes and may have a few methods.
Figure illustration below is an example of a gen/spec class diagram. The arrow points to the general class, or superclass. Often the lines connecting two or more subclasses to a superclass are joined using one arrow pointing to the superclass, but these could be shown as separate arrows as well. Notice that the top level is Person, representing any person. The attributes describe qualities that all people at a university have. The methods allow the class to change the name and the address (including telephone and email address). This is an abstract class, with no instances.
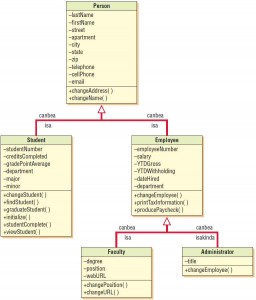
Student and Employee are subclasses, because they have different attributes and methods. An employee does not have a grade point average and a student does not have a salary. This is a simple version, and does not include employees that are students and students that work for the university. If these were added, they would be subclasses of the Employee and Student classes. Employee has two subclasses, Faculty and Administrator, because there are different attributes and methods for each of these specialized classes.
Subclasses have special verbs to define them. These are often run-on words, using isa for “is a,” isakinda for “is a kind of,” and canbea for “can be a.” There is no distinction between “is a” and “is an;” they both use isa.
isa | Faculty isa Employee |
isakinda | Administrator isakinda Employee |
canbea | Employee canbea Faculty |
Identifying Abstract Classes
You may be able to identify abstract classes by looking to see if a number of classes or database tables have the same elements, or if a number of classes have the same methods. You can create a general class by pulling out the common attributes and methods, or you might create a specialized class for the unique attributes and methods. Using a banking example, such as a withdrawal, a payment on a loan, or a check written, will all have the same method—they subtract money from the customer balance.
Finding Classes
There are a number of ways to determine classes. They may be discovered during interviewing or JAD sessions (described in Chapter 4), during facilitated team sessions, or from brainstorming sessions. Analyzing documents and memos may also reveal classes. One of the easiest ways is to use the CRC method described previously in this chapter. The analyst should also examine use cases, looking for nouns. Each noun may lead to a candidate, or potential, class. They are called candidate classes because some of the nouns may be attributes of a class.
Each class should exist for a distinct object that has a clear definition. Ask what the class knows, the attributes; and what the class knows how to do, the methods. Identify class relationships and the multiplicity for each end of the relationship. If the relationship is many-to-many, create an intersection or associative class, similar to the associative entity in an entity-relationship diagram.
Determining Class Methods
The analyst must determine class attributes and methods. Attributes are easy to identify, but the methods that work with the attributes may be more difficult. Some of the methods are standard, and are always associated with a class, such as new(), or the «create» method, which is an extension to UML created by a person or organization, called a stereotype. The « » symbols are not simply pairs of greater than and less than symbols, but are called guillemots or chevrons.
Another useful way to determine methods is to examine a CRUD matrix (see Chapter 7). Table below illustrates a CRUD matrix for course offerings. Each letter requires a different method. If there is a C for create, add a new() method. If there is a U for update, add an update() or change() method. If there is a D for delete, add a delete() or remove() method. If there is an R for read, add methods for finding, viewing, or printing. In the example shown, the textbook class would need a create method to add a textbook, and a read method to initiate a course inquiry, change a textbook, or find a textbook. If a textbook was replaced, an update method would be needed, and if a textbook was removed, a delete method would be required.
Activity | Department | Course | Textbook | Assignment | Exam |
---|---|---|---|---|---|
Add Department | C | ||||
View Department | R | ||||
Add Course | R | C | |||
Change Course | R | U | |||
Course Inquiry | R | R | R | R | R |
Add Textbook | R | R | C | ||
Change Textbook | R | RU | |||
Find Textbook | R | R | |||
Remove Textbook | R | D | |||
Add Assignment | R | C | |||
Change Assignment | R | RU | |||
View Assignment | R | R | |||
Add Exam | R | R | |||
Change Exam | R | RU | |||
View Exam | R | R |
Messages
In order to accomplish useful work, most classes need to communicate with one another. Information can be sent by an object in one class to an object in another class using a message, similar to a call in a traditional programming language. A message also acts as a command, telling the receiving class to do something. A message consists of the name of the method in the receiving class, as well as the attributes (parameters or arguments) that are passed with the method name. The receiving class must have a method corresponding to the message name.
Since messages are sent from one class to another, they may be thought of as an output or an input. The first class must supply the parameters included with the message and the second class uses the parameters. If a physical child data flow diagram exists for the problem domain, it may help to discover methods. The data flow from one primitive process to another represents the message, and the primitive processes should be examined as candidate methods.