Once the class diagram is drawn, it may be desirable to go back to the sequence diagram and include special symbols for each of the different types of classes introduced in the last section. Sequence diagrams in particular can be overbearing if an analyst doesn’t have a systematic approach to drawing them. The following steps are a useful approach to enhancing a sequence diagram:
- Include the actor from the use case diagram in the enhanced sequence diagram. This will be a stick figure from the use case diagram. There may be an additional actor on the right side of the diagram, such as a credit card company or bank.
- Define one or more interface classes for each actor. Each actor should have his or her own interface class.
- Create prototype Web pages for all human interfaces.
- Ensure each use case has one control class, although more may be created during the detailed design. Look for that control class and include it in the sequence diagram.
- Examine the use case to see what entity classes are present. Include these on the diagram.
- Realize that the sequence diagram may be modified again when doing detailed design, such as creating additional Web pages or control classes (one for each Web form submitted).
- To obtain a greater degree of reuse, consider moving methods from a control class to an entity class.
A Class Example for the Web
Classes may also be represented using special symbols for entity, boundary (or interface), and control classes. These are called stereotypes, an extension to UML, which are special symbols that may be used during analysis, but are often used when performing object-oriented design. They allow the analyst freedom to play with the design to optimize reusability.
The different types of classes are often used when working in the systems design phase. Figure below is an example illustrating a sequence diagram representing a student viewing his or her personal and course information. In the diagram, :View Student User Interface is an example of an interface class; :Student, :Section, and :Course are examples of entity classes; and :View Student Interface Controller and :Calculate Grade Point Average are control classes.
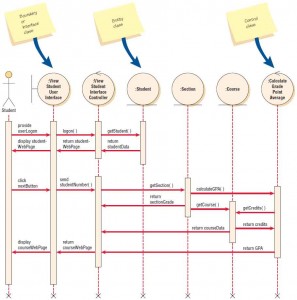
The student is shown on the left as an actor, and he or she provides a userLogon to the :View Student User Interface class. This is a Web form that obtains the student’s user ID and password. When the student clicks the Submit button, the Web form is passed to a :View Student Interface Controller. This class is responsible for the coordination of sending messages and receiving returned information from all the other classes.
The :View Student Interface Controller sends a getStudent( ) message to the :Student class, which reads a database table and proceeds to return the studentData.
The studentWebPage is returned to the :View Student User Interface, which displays the information in the Web browser. At the bottom of the page is a nextButton that the student clicks to view courses. When the user clicks this button, it sends a Web form to the :View Student Interface Controller. This form contains the studentNumber(), sent along with the studentWebPage, and is used to send a message to the :Section class to obtain the section grade. If the studentNumber() was not automatically sent, it would mean that the student would have to enter his or her studentNumber() again, which would not be a satisfactory user interface because it involves redundant keying. Notice that the :Student class is not involved, and that the focus of control (the vertical bar that is connected to the :Student class) ends before the second set of activities (the horizontal arrows pointing to the right) begins.
The :View Student Interface Controller class sends a getSection() message to the :Section class, which returns a sectionGrade. The :Section class also sends a calculateGPA() message to the :Calculate Grade Point Average class, which sends a message back to the :Course class. The :Course class returns the credits, which enables the :Calculate Grade Point Average class to determine the GPA and return it to the :View Student Interface Controller.
The :View Student Interface Controller would repeat sending messages to the :Section class until all sections for the student have been included. At this time, the :View Student Interface Controller would send the courseWebPage to the :View Student User Interface class, which would display the information in the browser.
Using the user interface, control, and entity classes also allows the analyst to explore and play with the design. The design mentioned previously would display all the student personal information on one page and the course information on a second page. The analyst may modify the design so that the student personal information and the course information appear on one Web page. These two possible scenarios would be reviewed with users to determine the best option. One of the difficulties for the analyst is to determine how to include the studentNumber after clicking the Next button, because the :Student class is no longer available. There are three ways to store and retransmit data from a Web page:
- Include the information in the URL displaying in the address or location area of the browser. In this case, the location line might read something like the following:
- http://www.cpu.edu/student/studentinq.html?studentNumber12345
- Everything after the question mark is data that may be used by the class methods. This means of storing data is easy to implement and is often used in search engines.
- There are several drawbacks to using this method, and the analyst must use due caution. The first concern is privacy—anyone can read the Web address. If the application involves medical information, credit card numbers, and so on, this is not a good choice. Most browsers will also display previous Web address data in subsequent sessions if the user enters the first few characters, and the information may be compromised, leading to identity theft. A second disadvantage is that the data are usually lost after the user closes the browser.
- Store the information in a cookie, a small file stored on the client (browser) computer. Cookies are the only way to store data that have persistence, existing beyond the current browser session. This enables the Web page to display a message such as “Welcome back, Robin. If you are not Robin, click here.” Cookies usually store primary key account numbers, but not credit card numbers or other private information. Cookies are limited to 20 per domain (such as www.cpu.edu) and each cookie must be 4,000 characters or less.
- The analyst must work with other business units to determine who needs to use cookies, and there must be some central control over the names used in the cookies. If the organization needs to have more than 20 cookies, a common solution is to create different domain names used by the organization, such as support.cpu.edu or instruction.cpu.edu.
- Use hidden Web form fields. These fields usually contain data that are sent by the server, are invisible, and do not occupy any space on the Web page. In the view student information example, the :View Student Interface Controller class added a hidden field containing the studentNumber to the studentWebPage form along with the nextButton. When the student clicks the nextButton, the studentNumber is sent to the server and the :View Student Interface Controller knows which student to obtain course and grade information for. The data in hidden forms is not saved from one browser session to another, so privacy is maintained.
Presentation, Business, and Persistence Layers in Sequence Diagrams
In the previous example, we showed all of the classes in the same diagram. When it comes to writing code for systems, it has been useful to look at sequence diagrams as having three distinct layers as follows:
- The presentation layer, which represents what the user sees. This layer contains the interface or boundary classes.
- The business layer, which contains the unique rules for this application. This layer contains the control classes.
- The persistence or data access layer, which describes obtaining and storing data. This layer contains the entity classes.
Ideally program code would be written separately for each of these layers.
With the introduction of Ajax, the lines became blurred. Ajax, an acronym for asynchronous JavaScript and XML, is a collection of techniques that allows Web applications to retrieve information from the server without altering the display of the current page. This turns out to be an advantage because the entire Web page does not need to be reloaded when it gets additional data from the server.
Before Ajax was created a user visiting aWeb site would answer some questions by entering data on aWeb-based form, then wait until a new page loaded. This was necessary because the code to validate, get the data, then answer the user resided on the server.With the advent of Ajax, the Web page is updated rapidly because much of the validation and other control logic is now included in the browser JavaScript code or on the client side. This means that business rules are included in both the boundary and the control class, so it might not be possible to have three distinct layers.