A stopwatch is a common tool used to measure the time between two events. Creating a stopwatch program in Java is a fantastic project for beginner to intermediate developers to hone their skills in programming, particularly in working with GUIs (Graphical User Interfaces), event handling, and threading. In this step-by-step tutorial, you’ll learn how to create a simple stopwatch program in Java from scratch.
Prerequisites
Before we start coding, ensure you have the following setup on your system:
- Java Development Kit (JDK): Ensure that JDK is installed on your machine. You can download it from Oracle’s official website.
- IDE or Text Editor: Use an IDE like IntelliJ IDEA, Eclipse, or NetBeans, or a text editor like VS Code or Notepad++.
Step 1: Setting Up the Project
The first step is to set up your project. Open your IDE or text editor and create a new Java project.
- Create a folder for your project (if not using an IDE).
- Inside this folder, create a file named
Stopwatch.java
. This will be our main program file.
Step 2: Plan the Program
Before jumping into the code, let’s break down the features we want in our stopwatch:
- A GUI with the following:
- A label to display the elapsed time.
- Three buttons: Start, Stop, and Reset.
- The ability to track time accurately.
- Proper threading to ensure the program remains responsive while running.
Step 3: Import Required Libraries
We need Java Swing for GUI components and some utility classes for managing time. Add the following imports at the top of the Stopwatch.java
file:
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
Code language: Java (java)
Step 4: Create the Stopwatch Class
Our program’s main class will be Stopwatch
, and it will extend JFrame
to represent a GUI window. Let’s set up the basic structure:
public class Stopwatch extends JFrame {
// Declare variables for time tracking
private long startTime;
private long elapsedTime;
private boolean running;
// Declare GUI components
private JLabel timeDisplay;
private JButton startButton;
private JButton stopButton;
private JButton resetButton;
public Stopwatch() {
// Constructor to initialize the GUI
setTitle("Stopwatch");
setSize(300, 200);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setLayout(new BorderLayout());
initComponents();
}
}
Code language: Java (java)
Step 5: Initialize GUI Components
Inside the Stopwatch
constructor, create a method initComponents()
to add and configure the GUI components.
private void initComponents() {
// Initialize components
timeDisplay = new JLabel("00:00:00", SwingConstants.CENTER);
timeDisplay.setFont(new Font("Arial", Font.BOLD, 30));
startButton = new JButton("Start");
stopButton = new JButton("Stop");
resetButton = new JButton("Reset");
// Add components to the frame
JPanel buttonPanel = new JPanel();
buttonPanel.setLayout(new GridLayout(1, 3));
buttonPanel.add(startButton);
buttonPanel.add(stopButton);
buttonPanel.add(resetButton);
add(timeDisplay, BorderLayout.CENTER);
add(buttonPanel, BorderLayout.SOUTH);
// Disable stop and reset buttons initially
stopButton.setEnabled(false);
resetButton.setEnabled(false);
}
Code language: Java (java)
This method initializes the components and lays them out using a simple grid layout for buttons and a central label for the time display.
Step 6: Add Functionality to Buttons
Now let’s add event listeners to our buttons. Update the Stopwatch
constructor to include calls to methods that will handle the button actions.
Start Button
The start button should begin the stopwatch. We use a separate thread to update the time without freezing the GUI.
private void startStopwatch() {
startButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
startTime = System.currentTimeMillis() - elapsedTime;
running = true;
// Enable/disable buttons
startButton.setEnabled(false);
stopButton.setEnabled(true);
resetButton.setEnabled(false);
// Create a new thread to update the time display
new Thread(() -> {
while (running) {
elapsedTime = System.currentTimeMillis() - startTime;
updateDisplay();
try {
Thread.sleep(100); // Update every 100ms
} catch (InterruptedException ex) {
ex.printStackTrace();
}
}
}).start();
}
});
}
Code language: Java (java)
Stop Button
The stop button pauses the stopwatch.
private void stopStopwatch() {
stopButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
running = false;
// Enable/disable buttons
startButton.setEnabled(true);
stopButton.setEnabled(false);
resetButton.setEnabled(true);
}
});
}
Code language: Java (java)
Reset Button
The reset button resets the stopwatch to its initial state.
private void resetStopwatch() {
resetButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
running = false;
elapsedTime = 0;
updateDisplay();
// Enable/disable buttons
startButton.setEnabled(true);
stopButton.setEnabled(false);
resetButton.setEnabled(false);
}
});
}
Code language: Java (java)
Step 7: Update the Display
The updateDisplay
method converts the elapsed time in milliseconds to a formatted string and updates the timeDisplay
label.
private void updateDisplay() {
int seconds = (int) (elapsedTime / 1000) % 60;
int minutes = (int) (elapsedTime / (1000 * 60)) % 60;
int hours = (int) (elapsedTime / (1000 * 60 * 60));
String timeString = String.format("%02d:%02d:%02d", hours, minutes, seconds);
timeDisplay.setText(timeString);
}
Code language: Java (java)
Step 8: Initialize the Button Actions
In the Stopwatch
constructor, call the methods to initialize the button actions:
public Stopwatch() {
setTitle("Stopwatch");
setSize(300, 200);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setLayout(new BorderLayout());
initComponents();
startStopwatch();
stopStopwatch();
resetStopwatch();
}
Code language: Java (java)
Step 9: Main Method to Launch the Program
Finally, create a main
method to run the program:
public static void main(String[] args) {
SwingUtilities.invokeLater(() -> {
Stopwatch stopwatch = new Stopwatch();
stopwatch.setVisible(true);
});
}
Code language: Java (java)
Complete Code
Here’s the complete code for the stopwatch program:
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class Stopwatch extends JFrame {
private long startTime;
private long elapsedTime;
private boolean running;
private JLabel timeDisplay;
private JButton startButton;
private JButton stopButton;
private JButton resetButton;
public Stopwatch() {
setTitle("Stopwatch");
setSize(300, 200);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setLayout(new BorderLayout());
initComponents();
startStopwatch();
stopStopwatch();
resetStopwatch();
}
private void initComponents() {
timeDisplay = new JLabel("00:00:00", SwingConstants.CENTER);
timeDisplay.setFont(new Font("Arial", Font.BOLD, 30));
startButton = new JButton("Start");
stopButton = new JButton("Stop");
resetButton = new JButton("Reset");
JPanel buttonPanel = new JPanel();
buttonPanel.setLayout(new GridLayout(1, 3));
buttonPanel.add(startButton);
buttonPanel.add(stopButton);
buttonPanel.add(resetButton);
add(timeDisplay, BorderLayout.CENTER);
add(buttonPanel, BorderLayout.SOUTH);
stopButton.setEnabled(false);
resetButton.setEnabled(false);
}
private void startStopwatch() {
startButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
startTime = System.currentTimeMillis() - elapsedTime;
running = true;
startButton.setEnabled(false);
stopButton.setEnabled(true);
resetButton.setEnabled(false);
new Thread(() -> {
while (running) {
elapsedTime = System.currentTimeMillis() - startTime;
updateDisplay();
try {
Thread.sleep(100);
} catch (InterruptedException ex) {
ex.printStackTrace();
}
}
}).start();
}
});
}
private void stopStopwatch() {
stopButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
running = false;
startButton.setEnabled(true);
stopButton.setEnabled(false);
resetButton.setEnabled(true);
}
});
}
private void resetStopwatch() {
resetButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e)
{
running = false;
elapsedTime = 0;
updateDisplay();
startButton.setEnabled(true);
stopButton.setEnabled(false);
resetButton.setEnabled(false);
}
});
}
private void updateDisplay() {
int seconds = (int) (elapsedTime / 1000) % 60;
int minutes = (int) (elapsedTime / (1000 * 60)) % 60;
int hours = (int) (elapsedTime / (1000 * 60 * 60));
String timeString = String.format("%02d:%02d:%02d", hours, minutes, seconds);
timeDisplay.setText(timeString);
}
public static void main(String[] args) {
SwingUtilities.invokeLater(() -> {
Stopwatch stopwatch = new Stopwatch();
stopwatch.setVisible(true);
});
}
}
Code language: Java (java)
Testing The Program
Open a Terminal or Command Prompt
Navigate to the directory where the Stopwatch.java
file is saved. For example:
- Windows: Open Command Prompt, then use the
cd
command:bashCopy codecd path\to\directory
- Mac/Linux: Open Terminal, then use the
cd
command:bashCopy codecd /path/to/directory
Compile the Code
Compile the Java program using the javac
command:
javac Stopwatch.java
Code language: Bash (bash)
- If the compilation is successful, it will generate a
Stopwatch.class
file in the same directory. - If there are errors, double-check the code for typos or syntax mistakes.
Run the Program
Run the compiled program using the java
command:
java Stopwatch
Code language: Java (java)
This will launch the stopwatch application with a GUI.
Using an IDE
If you’re using an IDE like IntelliJ IDEA, Eclipse, or NetBeans, follow these steps:
- Create a New Project:
- In your IDE, create a new Java project.
- Place the
Stopwatch.java
file in thesrc
directory of the project.
- Run the Program:
- Locate the
Stopwatch
class in the IDE. - Right-click on the file (or the class name) and choose Run.
- Locate the
Expected Behavior
Once the program runs, a window will open displaying:
- A large time label (
00:00:00
). - Three buttons: Start, Stop, and Reset.
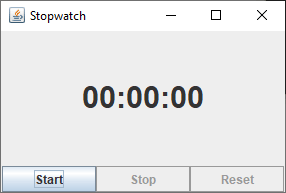
- Click Start to begin timing.
- Click Stop to pause.
- Click Reset to reset the timer.
Conclusion
You’ve now built a fully functional stopwatch program in Java! This project helped you practice:
- Using Swing to create GUIs.
- Handling button events with
ActionListener
. - Implementing threading for time updates.
Feel free to expand this program by adding features such as lap timers or exporting results to a file.